Primefaces Spring Boot Hello World Example – Primefaces Tutorial
Hello and welcome again to elgarnaoui.com, in this tutorials we will create a Hello world using spring boot and JSF primefaces framework.
So let’s start :
Tools used in this tutorial :
- Eclipse 2020-03 or IntelliJ IDEA Community Edition 2020.2
- Spring boot 2.3.2.RELEASE
- Java 8
- Primefaces 5.1
- Myfaces-impl 2.2.6
Let’s create our project :
In eclipse click new > other > Spring Starter Project and click next then choose the name of your project, group, artifact and version :
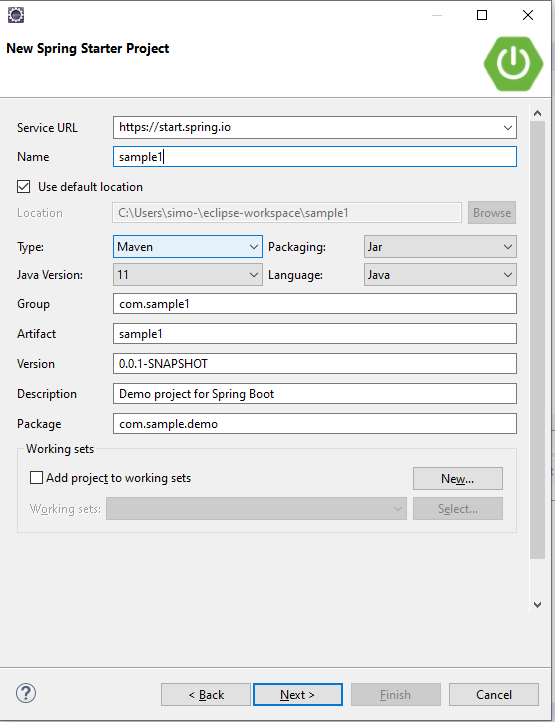
Next shoose the spring boot version and other dependencies like devtools :
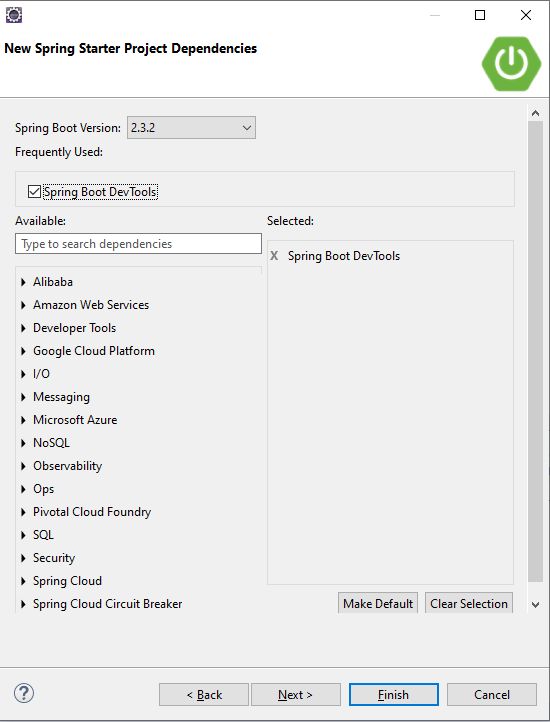
Click Finish, then the project is created now.
Project Structure :
This is the web project structure build using Maven.
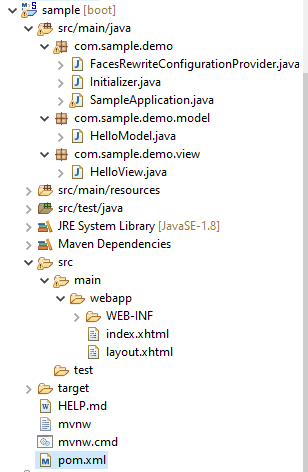
Maven dependencies :
In pom.xml
file of your maven project, add the dependencies below.
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.3.2.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.sample</groupId>
<artifactId>sample</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>sample</name>
<description>Demo project for Spring Boot</description>
<packaging>jar</packaging>
<properties>
<java.version>8</java.version>
</properties>
<!-- Add typical dependencies for a web application -->
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.apache.myfaces.core</groupId>
<artifactId>myfaces-impl</artifactId>
<version>2.2.6</version>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>org.apache.myfaces.core</groupId>
<artifactId>myfaces-api</artifactId>
<version>2.2.6</version>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>org.apache.tomcat.embed</groupId>
<artifactId>tomcat-embed-core</artifactId>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>org.apache.tomcat.embed</groupId>
<artifactId>tomcat-embed-jasper</artifactId>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>org.primefaces</groupId>
<artifactId>primefaces</artifactId>
<version>5.1</version>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>org.primefaces.extensions</groupId>
<artifactId>all-themes</artifactId>
<version>1.0.8</version>
</dependency>
<dependency>
<groupId>org.ocpsoft.rewrite</groupId>
<artifactId>rewrite-servlet</artifactId>
<version>2.0.12.Final</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<!-- Package as an executable jar -->
<build>
<outputDirectory>src/main/webapp/WEB-INF/classes</outputDirectory>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
Model class :
In package model create a managed bean java class HelloModel.java
with these annotations from JSF to make this class a ManagedBean
@ManagedBean(name = "model", eager = true)
@SessionScoped
HelloModel.java as below :
package com.sample.demo.model;
import javax.faces.bean.ManagedBean;
import javax.faces.bean.SessionScoped;
/**
* Created by elgarnaoui.com.
*/
@ManagedBean(name = "model", eager = true)
@SessionScoped
public class HelloModel {
private String hello = "Hello /";
private String world = "World/";
public String getHello() {
return hello;
}
public void setHello(String hello) {
this.hello = hello;
}
public String getWorld() {
return world;
}
public void setWorld(String world) {
this.world = world;
}
}
View Class :
In view package create a HelloView.java
class extends from the ManagedBean java class HelloWorld.java
as below :
package com.sample.demo.view;
import javax.faces.bean.ManagedBean;
import javax.faces.bean.RequestScoped;
import com.sample.demo.model.HelloModel;
/**
* Created by elgarnaoui.com.
*/
@ManagedBean(name = "hello", eager = true)
@RequestScoped
public class HelloView extends HelloModel{
public HelloView() {
super();
// TODO Auto-generated constructor stub
}
}
Demo package :
In this package create FacesRewriteConfigurationProvider.java
and Initializer.java
and update your SampleAppliction.java
as below :
SampleAppliction.java
package com.sample.demo;
import java.util.EnumSet;
import javax.faces.webapp.FacesServlet;
import javax.servlet.DispatcherType;
import org.ocpsoft.rewrite.servlet.RewriteFilter;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.EnableAutoConfiguration;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.boot.builder.SpringApplicationBuilder;
import org.springframework.boot.web.servlet.FilterRegistrationBean;
import org.springframework.boot.web.servlet.ServletRegistrationBean;
import org.springframework.boot.web.servlet.support.SpringBootServletInitializer;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.ComponentScan;
/**
* Created by elgarnaoui.com.
*/
@SpringBootApplication
@EnableAutoConfiguration
@ComponentScan({"com.sample.demo"})
public class SampleApplication extends SpringBootServletInitializer{
public static void main(String[] args) {
SpringApplication.run(SampleApplication.class, args);
}
@Override
protected SpringApplicationBuilder configure(SpringApplicationBuilder application) {
return application.sources(SampleApplication.class, Initializer.class);
}
@SuppressWarnings("rawtypes")
@Bean
public ServletRegistrationBean servletRegistrationBean() {
FacesServlet servlet = new FacesServlet();
ServletRegistrationBean servletRegistrationBean = new ServletRegistrationBean(servlet, "*.jsf");
return servletRegistrationBean;
}
@SuppressWarnings("unchecked")
@Bean
public FilterRegistrationBean rewriteFilter() {
FilterRegistrationBean rwFilter = new FilterRegistrationBean(new RewriteFilter());
rwFilter.setDispatcherTypes(EnumSet.of(DispatcherType.FORWARD, DispatcherType.REQUEST,
DispatcherType.ASYNC, DispatcherType.ERROR));
rwFilter.addUrlPatterns("/*");
return rwFilter;
}
}
FacesRewriteConfigurationProvider.java
package com.sample.demo;
import javax.servlet.ServletContext;
import org.ocpsoft.rewrite.annotation.RewriteConfiguration;
import org.ocpsoft.rewrite.config.Configuration;
import org.ocpsoft.rewrite.config.ConfigurationBuilder;
import org.ocpsoft.rewrite.servlet.config.HttpConfigurationProvider;
import org.ocpsoft.rewrite.servlet.config.rule.Join;
/**
* Created by elgarnaoui.com.
*/
@RewriteConfiguration
public class FacesRewriteConfigurationProvider extends HttpConfigurationProvider {
@Override
public int priority()
{
return 10;
}
@Override
public Configuration getConfiguration(final ServletContext context)
{
return ConfigurationBuilder.begin()
.addRule(Join.path("/sample/").to("/index.jsf"))
.addRule(Join.path("/sample/hello/").to("/index.jsf"));
}
}
Initializer.java
package com.sample.demo;
import org.springframework.context.annotation.Configuration;
import javax.servlet.ServletContext;
import javax.servlet.ServletException;
/**
* Created by elgarnaoui.com.
*/
@Configuration
public class Initializer implements org.springframework.boot.web.servlet.ServletContextInitializer {
@Override
public void onStartup(ServletContext servletContext) throws ServletException {
System.err.println("------------------------------------");
servletContext.setInitParameter("primefaces.CLIENT_SIDE_VALIDATION", "true");
servletContext.setInitParameter("primefaces.THEME", "bootstrap");
}
}
Webapp folder :
In this folder create 2 files.xhtml with taglibs from JSF, JSF core, Facelets and PrimeFaces:
layout.xhtml
as below :
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://xmlns.jcp.org/jsf/html"
xmlns:ui="http://xmlns.jcp.org/jsf/facelets"
xmlns:f="http://xmlns.jcp.org/jsf/core"
xmlns:p="http://primefaces.org/ui">
<f:view>
<h:head>
<title><ui:insert name="title">Default title</ui:insert></title>
</h:head>
<h:body>
<div class="ui-grid ui-grid-responsive">
<div class="ui-grid-row">
<div class="ui-grid-col-3"></div>
<div class="ui-grid-col-6"></div>
<div class="ui-grid-col-3"></div>
</div>
<div class="ui-grid-row">
<div class="ui-grid-col-3"></div>
<div class="ui-grid-col-6">
<p:growl for="errors" showDetail="true" autoUpdate="true"/>
<ui:insert name="content">Default content</ui:insert>
</div>
<div class="ui-grid-col-3"></div>
</div>
</div>
</h:body>
</f:view>
</html>
index.xhtml as below :
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://xmlns.jcp.org/jsf/html"
xmlns:ui="http://xmlns.jcp.org/jsf/facelets"
xmlns:f="http://xmlns.jcp.org/jsf/core"
xmlns:p="http://primefaces.org/ui" >
<ui:composition template="layout.xhtml">
<ui:define name="content">
<p:panelGrid columns="2">
<h:outputText value="#{hello.hello}"/>
<h:outputText value="#{hello.world}"/>
</p:panelGrid>
</ui:define>
</ui:composition>
</html>
Run Application :
Now go to SampleApplication.java > right click >Run As > Java Application
and when the tomcat running go to your browser and type http://localhost:8080/sample/hello/ or http://localhost:8080/sample/ as your config in FacesRewriteConfigurationProvider.java
and you will get a hello world as :

Thank you for reading this post, to support us share it on social media, and if you have any issues, request or question let me know in the comment section đŸ™‚
Code source :