Hello World Your First Java 14 Program
To learn a new programming language we start usually with a Hello World program. So let’s create our Java Hello World program.
Requirements:
- JDK 14 installed.
- JAVA_HOME environment variable configured.
PS: Refer to this link to download JDK 14 and to this one to install and configure the environment variable
We can simplify the Java Hello world program process into three steps:
- Create the program by typing it into a text editor and saving it to a file – HelloWorld.java.
- Compile it by typing “javac HelloWorld.java” in the terminal window.
- Run it by typing “java HelloWorld” in the terminal window.
The program below is the simplest Java program for printing “Hello World” to the screen.
/* This is an example of a hello world program in Java.
File name: "HelloWorld.java". */
class HelloWorld {
// Your program starts within the main function().
// Write "Hello, World" in the window terminal.
public static void main(String args[]) {
System.out.println("Hello, World");
}
}
How to run this program with Java command:
First, add this code to a file with a HelloWorld.java
name, then open a command line and position it to the folder where your HelloWorld.java exists and run this command:
Javac HelloWorld.java

After running this javac command you will find in your folder one other file HelloWorld.class
.
Then, run java HelloWorld
command and you will see you Hello, World
message in the command line:

output: Hello, World
If you get this message: javac' is not recognized as an internal or external command, operable program or batch file.
Try this from StackOverflow:
- Find the Java path; it looks like this:Â
C:\Program Files\Java\jdkxxxx\bin\
- Start-menu search for “environment variable” to open the options dialog.
- ExamineÂ
PATH
. Remove old Java paths. - Add the new Java path toÂ
PATH
. - EditÂ
JAVA_HOME
. - Close and re-open console/IDE.
If you still get this error or other, Ask me in the comment below.
Java Hello World Program using IntelliJ IDEA:
To create a Hello World program using Intellij Idea Community or Eclipse, download and install one. Then lunch it.
Click New Project and then choose java and JDK 14 or another version of JDK then click next:
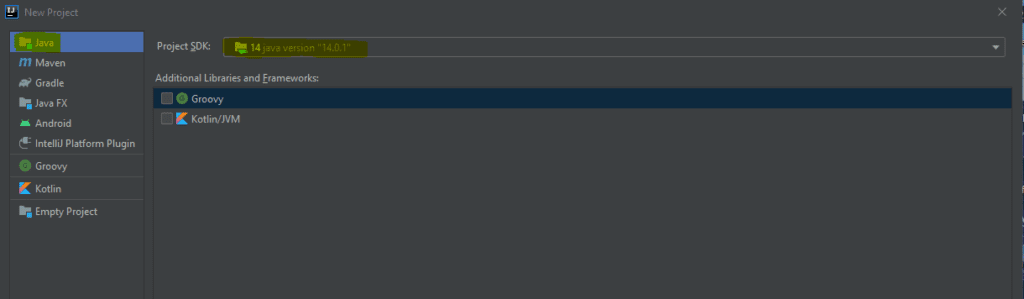
After that click next and another next, Type your project name for example HelloWorld
then click finish.
You will get this window when you click finish:

Go the src
and right click > New > Java Class and type the name of your Java for example HelloWorld
and double click. Your class should look like that:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World");
}
}
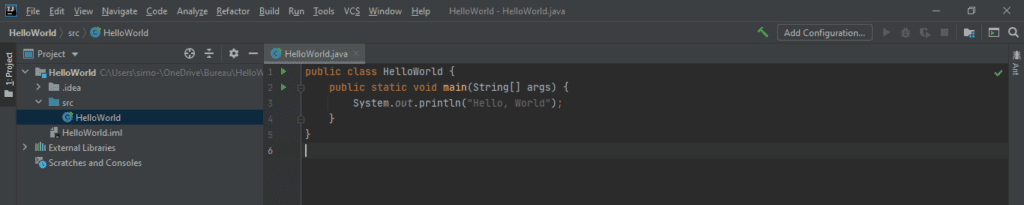
Then right click in your Java class and Run 'HelloWorld.main()'
and you will see your Hello, World
message
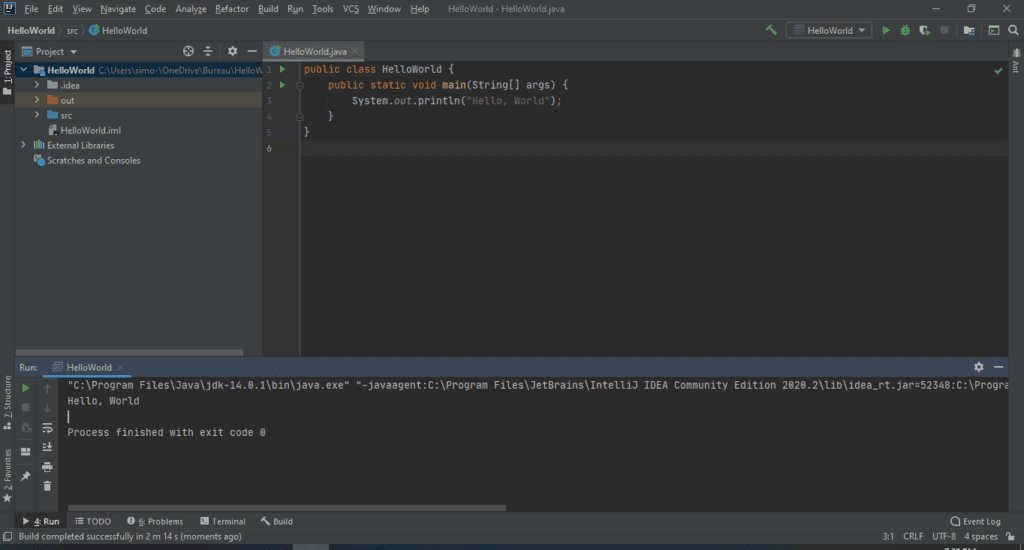
To support us share this post with your friends on social media.
If you encounter any problem or have any question, let me know in the comment section below
Code Source: