NullPointerException in Java
Introduction:
A NullPointerException is a runtime exception in Java that occurs when you try to use a reference variable that is null. In other words, it’s an exception that is thrown when you try to access a member of an object that doesn’t exist. NullPointerException is a subclass of RuntimeException, which means that it’s an unchecked exception that you don’t need to declare in your method’s throws clause.
Causes of NullPointerException:
The most common causes of NullPointerException are:
- Calling a method or accessing a field on a null object reference.
- Accessing an element of a null array.
- Throwing null as if it were an Throwable value.
- Calling a static method on a null class reference.
- Accessing a static field on a null class reference.
- Trying to synchronize on a null object reference.
Example 1: Calling a Method on a Null Object Reference
Here’s an example that demonstrates how a NullPointerException can occur when calling a method on a null object reference:
package elgarnaoui.com;
public class Main {
public static void main(String[] args) {
String str = null;
System.out.println(str.length()); // This will throw a NullPointerException
}
}
In the above code, we’re trying to call the length()
method on a null object reference, which will throw a NullPointerException:
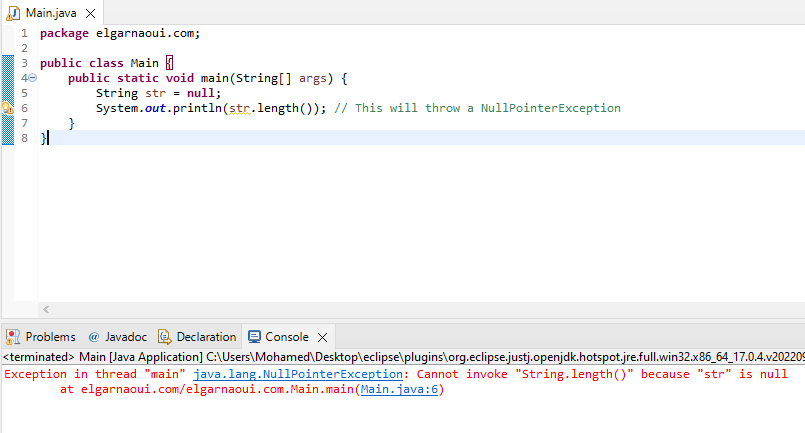
Example 2: Accessing an Element of a Null Array
Here’s an example that demonstrates how a NullPointerException can occur when accessing an element of a null array:
package elgarnaoui.com;
public class Main {
public static void main(String[] args) {
int[] arr = null;
System.out.println(arr[0]); // This will throw a NullPointerException
}
}
In the above code, we’re trying to access the first element of a null array, which will throw a NullPointerException:
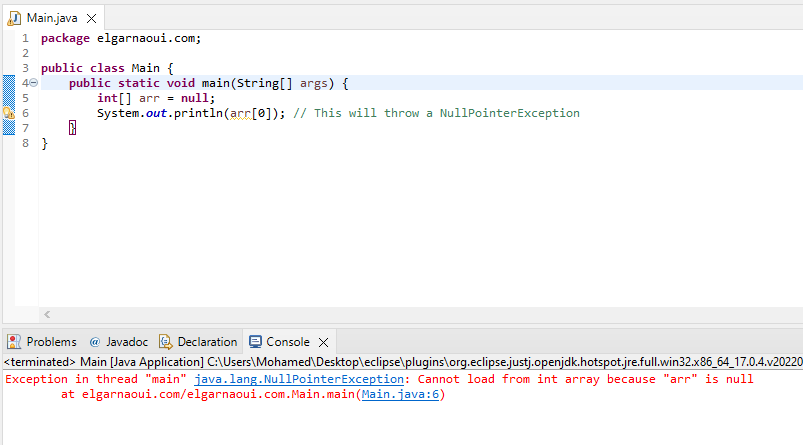
Example 3: Throwing Null as if it Were an Throwable Value
Here’s an example that demonstrates how a NullPointerException can occur when throwing null as if it were an Throwable value:
package elgarnaoui.com;
public class Main {
public static void main(String[] args) throws Exception {
throw null; // This will throw a NullPointerException
}
}
In the above code, we’re trying to throw null as if it were an Throwable value, which will throw a NullPointerException:
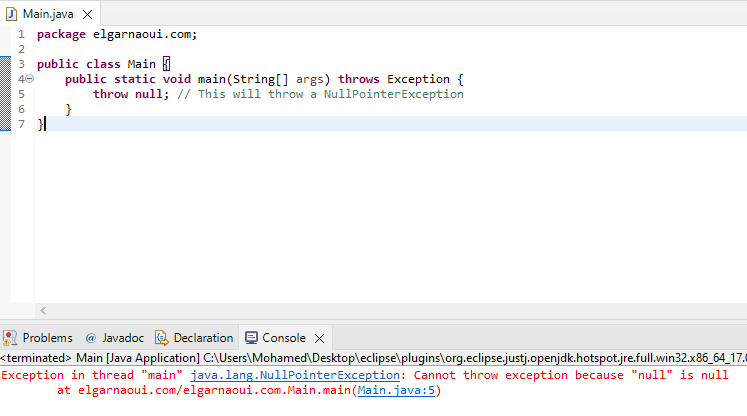
How to Fix NullPointerException:
To fix a NullPointerException, you need to find out why the object is null and make sure it’s properly initialized before you try to use it. Here are some ways to fix a NullPointerException:
- Initialize the object before using it.
- Check if the object is null before using it.
- Use try-catch blocks to handle NullPointerExceptions.
- Use null-safe methods and operators, such as Optional and Elvis operator in Java 8 and later.
- Use assertions to check for null values.
Example 4: Checking if an Object is Null Before Using It
Here’s an example that demonstrates how to check if an object is null before using it:
package elgarnaoui.com;
public class Main {
public static void main(String[] args) {
String str = null;
if (str != null) {
System.out.println(str.length());
} else {
System.out.println("str is null");
}
}
}
In the above code, we’re checking if str
is null before calling the length()
method, which will prevent a NullPointerException from occurring.
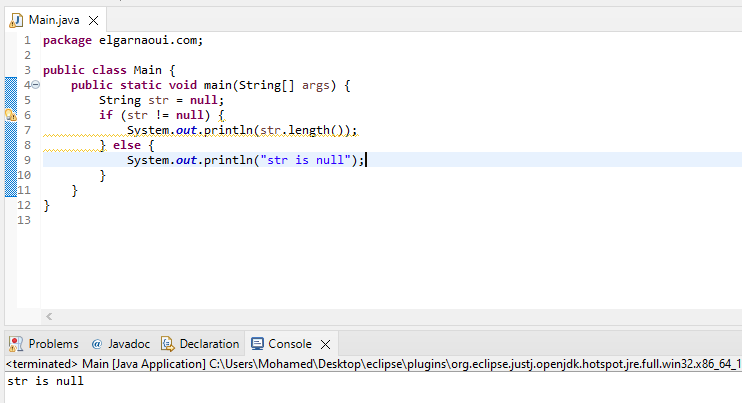
Example 5: Using Null-Safe Methods and Operators
Here’s an example that demonstrates how to use null-safe methods and operators in Java 8 and later:
package elgarnaoui.com;
import java.util.Optional;
public class Main {
public static void main(String[] args) {
String str = null;
Optional.ofNullable(str)
.ifPresent(s -> System.out.println(s.length()));
}
}
In the above code, we’re using the Optional.ofNullable()
method to create an Optional object that wraps str
. Then we’re using the ifPresent()
method to call the length()
method on str
only if it’s not null.
Best Practices:
Here are some best practices to avoid NullPointerExceptions:
- Always initialize your objects before using them.
- Use try-catch blocks to handle NullPointerExceptions.
- Use null-safe methods and operators, such as Optional and Elvis operator in Java 8 and later.
- Use static code analysis tools, such as SonarQube and FindBugs, to detect potential NullPointerExceptions.
- Use assertions to check for null values.
- Document your methods to indicate whether they can return null values.
Conclusion:
NullPointerException is a common runtime exception in Java that can be easily avoided by properly initializing your objects and checking for null values. By following best practices and using null-safe methods and operators, you can write more robust and reliable code.
That’s it! You’ve just learned more about NullPointerExceptions in Java with code examples.
Let’s level up our Java skills together! join our community (Elgarnaoui.com) and share this post on social media! #Java #Programming #DeveloperCommunity