Chat room application using Java
In this tutorial, we will make a chat room application using java swing, socket, and threads on the local network.
Overview:
This video is a demo of what we will develop in this chat room application tutorial using Java Socket:
TCP Protocol:
First, we need to know what is a TCP protocol?
TCP is a protocol that allows a point-to-point connection between two applications. It is a reliable protocol that guarantees receipt in order of sending data.
TCP uses the notion of the port to allow several applications to use this same protocol.
In a link between two computers, one of the two acts as a server and the other as a client.
ServerSocket Java Class:
The ServerSocket class is used on the server-side: it simply waits for calls from the client (s). It is an object of the Socket type which supports the transmission of data.
This class represents the server part of the socket. An object of this class is associated with a port on which it will wait for connections from a client. Usually, when a connection request arrives, a thread is started to ensure dialogue with the client without blocking connections from other clients.
The ServerSocket class has several constructors, the main ones being:
Constructor | Role |
ServerSocket() | Default Constructor |
ServerSocket(int) | Create a socket on the port provided as a parameter |
ServerSocket(int, int) | Create a socket on the port and with the maximum size of the file provided in parameters |
The ServerSocket class constructors can throw an exception of type IOException and has several methods:
Method | Role |
Socket accept() | wait fora new connection |
void close() | close the socket |
If a client program tries to communicate with the server, the accept () method returns a socket that encapsulates communication with that client.
Example:
ServerSocket socketServer = new ServerSocket(1234);
//create a socket server
Socket socketClient = socketServer.accept();
//connection with a client socket
Socket class:
Sockets implement the Transmission Control Protocol (TCP). The class contains the methods for creating the corresponding input and output streams. Sockets are the basis of network communications.
The Socket class has several constructors, the main ones being:
Constructor | Role |
Socket() | default Constructor |
Socket(String, int) | Create a socket on the machine whose name and port are provided in parameters |
Socket(InetAddress, int) | Create a socket on the machine whose IP address and port are provided as parameters |
The Socket class has many methods, the main ones being:
Method | Role |
InetAddress getInetAddress() | Returns the I.P. address to which the socket is connected |
void close() | Close the socket |
InputStream getInputStream() | Return an input stream to receive data from the socket |
OutputStream getOutputStream() | Return an output stream to emit socket data |
int getPort() | Returns the port used by the socket |
Example:
InetAddress server = InetAddress.getByName("string");
socket = new Socket(server, intport);
To learn more about Socket and ServerSocket refer the Java documentation.
Create Chat room application:
Now, after you understand the TCP protocol and Socket classes, let’s create our chat room application.
Projects structure:
This is the ChatRoom and ClientChatRoom project structure:
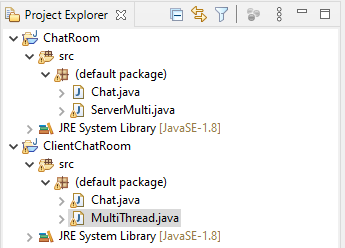
Chat room application Server side:
Create a java project named Chatroom, and create a class Chat.java
extends from the JFrame as follow:
import java.awt.EventQueue;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.border.EmptyBorder;
import javax.swing.JLabel;
import java.io.IOException;
import java.net.ServerSocket;
import java.net.Socket;
import java.awt.List;
public class Chat extends JFrame {
/**
*
*/
private static final long serialVersionUID = 1L;
private JPanel contentPane;
private JLabel lblWaitForConnection;
private List list ;
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
Chat frame = new Chat();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the frame.
*/
public Chat() {
new Thread(new Runnable()
{
public void run() {
try (ServerSocket serverSocket = new ServerSocket(1234);){
while(true)
{
Socket c = serverSocket.accept() ;
lblWaitForConnection.setText(c.getRemoteSocketAddress().toString());
System.out.println("start a connection ... ");
(new ServerMulti(c,list)).start();
}
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}).start();
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 450, 300);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
setContentPane(contentPane);
contentPane.setLayout(null);
lblWaitForConnection = new JLabel("Waiting for a connection ...");
lblWaitForConnection.setBounds(31, 11, 368, 14);
contentPane.add(lblWaitForConnection);
list = new List();
list.setBounds(31, 31, 326, 194);
contentPane.add(list);
}
}
Then, create a class ServerMulti.java
:
import java.awt.List;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.io.PrintWriter;
import java.net.Socket;
public class ServerMulti extends Thread {
private Socket sc ;
private List list ;
public ServerMulti(Socket sc,List l) {
// TODO Auto-generated constructor stub
this.sc = sc ;
list = l ;
}
@Override
public void run() {
System.out.println("lunch the server Multi ");
BufferedReader br = null ;
PrintWriter pw ;
try {
InputStream is = sc.getInputStream();
InputStreamReader isr = new InputStreamReader(is);
br = new BufferedReader(isr);
//OUT
OutputStream io = sc.getOutputStream() ;
pw = new PrintWriter(io,true);
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
while(true)
{
try {
System.out.println("waiting for messages ... ");
String msg = br.readLine() ;
System.out.println(msg);
list.add(msg);
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
}
Those classes are for the server-side, now we will create our client-side.
Chat room Application Client side:
Create another Java project ClientChatRoom
, then create a Chat.java
class:
import java.awt.EventQueue;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.border.EmptyBorder;
import javax.swing.JTextField;
import javax.swing.JLabel;
import javax.swing.JButton;
import java.awt.event.ActionListener;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.io.PrintWriter;
import java.net.Socket;
import java.net.UnknownHostException;
import java.awt.event.ActionEvent;
public class Chat extends JFrame {
/**
*
*/
private static final long serialVersionUID = 1L;
private JPanel contentPane;
private JTextField textField;
private JTextField textField_1;
private JTextField textField_2;
private JButton btnSend;
private BufferedReader br ;
private PrintWriter pw ;
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
Chat frame = new Chat();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the frame.
*/
public Chat() {
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 300, 242);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
setContentPane(contentPane);
contentPane.setLayout(null);
textField = new JTextField();
textField.setBounds(135, 10, 86, 20);
contentPane.add(textField);
textField.setColumns(10);
textField_1 = new JTextField();
textField_1.setBounds(135, 41, 86, 20);
contentPane.add(textField_1);
textField_1.setColumns(10);
JLabel lblIp = new JLabel("IP");
lblIp.setBounds(33, 13, 46, 14);
contentPane.add(lblIp);
JLabel lblPort = new JLabel("Port");
lblPort.setBounds(33, 44, 46, 14);
contentPane.add(lblPort);
JButton btnConnecter = new JButton("Connected");
btnConnecter.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
String IP = textField.getText() ;
int PORT = Integer.parseInt(textField_1.getText());
Socket sc = null ;
try {
sc = new Socket(IP, PORT);
} catch (UnknownHostException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
try {
//IN
InputStream is = sc.getInputStream() ;
InputStreamReader isr = new InputStreamReader(is);
br = new BufferedReader(isr);
//OUT
OutputStream io = sc.getOutputStream() ;
pw = new PrintWriter(io,true);
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
btnConnecter.setEnabled(false);
}
});
btnConnecter.setBounds(132, 73, 89, 23);
contentPane.add(btnConnecter);
textField_2 = new JTextField();
textField_2.setBounds(10, 127, 252, 20);
contentPane.add(textField_2);
textField_2.setColumns(10);
btnSend = new JButton("Send");
btnSend.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
pw.println(textField_2.getText()) ;
textField_2.setText("");
}
});
btnSend.setBounds(171, 158, 89, 23);
contentPane.add(btnSend);
}
}
And, Create another class MultiThread.java
:
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.io.PrintWriter;
import java.net.Socket;
public class MultiThread extends Thread {
private Socket sc ;
public MultiThread(Socket sc) {
// TODO Auto-generated constructor stub
this.sc = sc ;
}
@Override
public void run() {
BufferedReader br = null ;
PrintWriter pw ;
try {
InputStream is = sc.getInputStream();
InputStreamReader isr = new InputStreamReader(is);
br = new BufferedReader(isr);
//OUT
OutputStream io = sc.getOutputStream() ;
pw = new PrintWriter(io,true);
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
while(true)
{
try {
String msg = br.readLine() ;
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
}
Alright, now everything is done you need just to run it, To do that refer to the video in the Overview section.
Thanks for being here, if you have encountered any problems let us know in comment section, and if you would like to support us subscribe, follow and share this post with your friends on social media.