How To Create A Tic Tac Toe Game In Android
In this tutorial, we will explain how to create a Tic Tac Toe game in android using java.
Tic Tac Toe game overview:
This the overview on the tablet when we test our Tic Tac Toe game in android:




Project Structure:
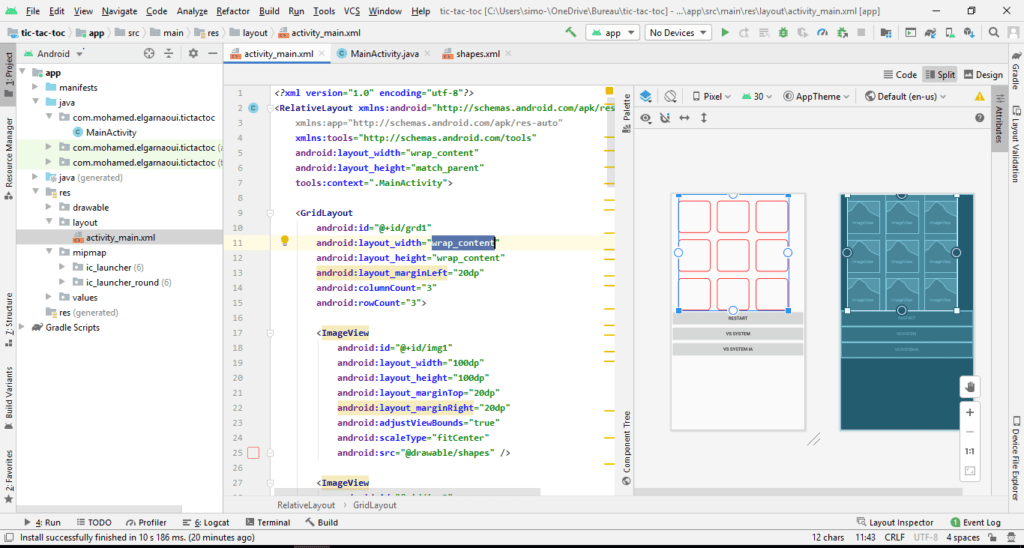
XML Layout:
Let’s start by creating our graphic interface using android studio and XML, As you noticed before we have just one graphic interface that contains 3 buttons for restart, play vs computer, and play vs computer artificial intelligence AI and the nine imageView
for the X and 0.
So the activity_main.xml
is:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="wrap_content"
android:layout_height="match_parent"
tools:context=".MainActivity">
<GridLayout
android:id="@+id/grd1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="20dp"
android:columnCount="3"
android:rowCount="3">
<ImageView
android:id="@+id/img1"
android:layout_width="100dp"
android:layout_height="100dp"
android:layout_marginTop="20dp"
android:layout_marginRight="20dp"
android:adjustViewBounds="true"
android:scaleType="fitCenter"
android:src="@drawable/shapes" />
<ImageView
android:id="@+id/img2"
android:layout_width="100dp"
android:layout_height="100dp"
android:layout_marginTop="20dp"
android:layout_marginRight="20dp"
android:src="@drawable/shapes" />
<ImageView
android:id="@+id/img3"
android:layout_width="100dp"
android:layout_height="100dp"
android:layout_marginTop="20dp"
android:src="@drawable/shapes" />
<ImageView
android:id="@+id/img4"
android:layout_width="100dp"
android:layout_height="100dp"
android:layout_marginTop="20dp"
android:layout_marginRight="20dp"
android:src="@drawable/shapes" />
<ImageView
android:id="@+id/img5"
android:layout_width="100dp"
android:layout_height="100dp"
android:layout_marginTop="20dp"
android:layout_marginRight="20dp"
android:src="@drawable/shapes" />
<ImageView
android:id="@+id/img6"
android:layout_width="100dp"
android:layout_height="100dp"
android:layout_marginTop="20dp"
android:src="@drawable/shapes" />
<ImageView
android:id="@+id/img7"
android:layout_width="100dp"
android:layout_height="100dp"
android:layout_marginTop="20dp"
android:layout_marginRight="20dp"
android:src="@drawable/shapes" />
<ImageView
android:id="@+id/img8"
android:layout_width="100dp"
android:layout_height="100dp"
android:layout_marginTop="20dp"
android:layout_marginRight="20dp"
android:src="@drawable/shapes" />
<ImageView
android:id="@+id/img9"
android:layout_width="100dp"
android:layout_height="100dp"
android:layout_marginTop="20dp"
android:src="@drawable/shapes" />
</GridLayout>
<Button
android:id="@+id/brestart"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@+id/grd1"
android:onClick="restart"
android:text="Restart" />
<Button
android:id="@+id/btnSystem"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@+id/brestart"
android:onClick="changeMode"
android:text="Vs System" />
<Button
android:id="@+id/btnSystem1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@+id/btnSystem"
android:onClick="changeMode"
android:text="Vs System IA" />
</RelativeLayout>
After creating the graphic design now let’s move to the algorithm and concept to make this game work as expected.
MainActivity.java
:
The Main activity class will have multi-functions and algorithms to interact with the computer and make the Tic Tac Toe game work perfectly.
Then, we will create this list of function to make the Tic Tac Toe works, So let’s start by defining all the functions needed in our game:
init
()setVirtualMatrix(View v)
randomAffectation()
gameOver()
systemChoice()
systemAffect(int a, char c)
restart(View v)
changeMode(View view)
.
Those are the functions that we will use to create our Tic Tac Toe game in android using java and xml.
The main activity class it will look like:
package com.mohamed.elgarnaoui.tictactoc;
import android.content.DialogInterface;
import android.support.v7.app.AlertDialog;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.ImageView;
import android.widget.Toast;
import java.util.Random;
public class MainActivity extends AppCompatActivity implements View.OnClickListener {
Random rd = new Random();
String game_mode = "multi/";
int[][] virtualM = new int[3][3];
ImageView[][] images = new ImageView[3][3];
Button bsystem,bsystem1 ;
boolean player = true ; //player 1 = true , player 2 = false
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
@Override
protected void onStart() {
super.onStart();
init();
}
private void init()
{
bsystem = (Button) findViewById(R.id.btnSystem);
bsystem1 = (Button) findViewById(R.id.btnSystem1);
int k = 1 ;
for(int i=0;i<3;i++)
for(int j=0;j<3;j++)
{
images[i][j] = (ImageView) findViewById
(getResources().getIdentifier
("img"+k,"id",getPackageName()));
k++;
}
for(int i=0;i<3;i++)
for(int j=0;j<3;j++)
images[i][j].setOnClickListener(this);
}
private void setVirtualMatrix(View v)
{
for(int i=0;i<3;i++)
for(int j=0; j<3; j++)
{
if(images[i][j].getId() == v.getId())
{
if(virtualM[i][j]==0)
{
if (player)
{
images[i][j].setImageResource(R.drawable.red_x);
virtualM[i][j] = 1;
if(game_mode.equals("multi"))
player = false;
else if(game_mode.equals("vsSystem"))
{
if(gameOver()==0) {
randomAffectation();
}
}
else
{
if(gameOver()==0)
{
systemChoice();
}
}
}
else
{
images[i][j].setImageResource(R.drawable.red_0);
virtualM[i][j] = -1;
player = true;
}
}
}
}
}
private void randomAffectation()
{
int a = -1, b = -1;
do {
a = rd.nextInt(3);
b = rd.nextInt(3);
} while (virtualM[a][b] != 0);
images[a][b].setImageResource(R.drawable.red_0);
virtualM[a][b] = -1;
}
private int gameOver()
{
boolean test = false ;
int sl,sc,sd1=0,sd2=0;
for(int i=0;i<3;i++)
{
sl = 0;
sc = 0;
for(int j=0;j<3;j++)
{
sl+= virtualM[i][j];
sc+= virtualM[j][i];
if(i==j)
sd1+=virtualM[i][j];
if(i+j==2)
sd2+=virtualM[i][j];
if(virtualM[i][j] == 0)
test = true ;
}
if(sl==3 || sc ==3)
return 1;
if(sl==-3 || sc == -3)
return 2;
}
if(sd1==3 || sd2 == 3)
return 1;
if (sd1 ==-3 || sd2 == -3)
return 2;
if(!test)
return 3;
return 0;
}
private void systemChoice()
{
boolean test = false ;
int sl,sc,sd1=0,sd2=0;
for(int i=0;i<3;i++)
{
sl = 0;
sc = 0;
for(int j=0;j<3;j++)
{
sl+= virtualM[i][j];
sc+= virtualM[j][i];
if(i==j)
sd1+=virtualM[i][j];
if(i+j==2)
sd2+=virtualM[i][j];
}
if(sl==-2 || sl == 2)
{
systemAffect(i,'l');
test = true ;
}
else if(sc==-2 || sc==2)
{
systemAffect(i,'c');
test = true ;
}
}
if (!test && (sd1 ==-2 || sd1 == 2)) {
systemAffect(-1, 'd');
test = true ;
}
if(!test && (sd2 == -2 || sd2 == 2)) {
systemAffect(-1, 'w');
test = true;
}
if(!test)
randomAffectation();
}
private void systemAffect(int a, char c)
{
boolean test = false ;
for(int i=0;i<3;i++)
{
for(int j=0;j<3;j++)
{
if(i==a)
{
if(c=='l' && virtualM[i][j]==0) {
images[i][j].setImageResource(R.drawable.red_0);
virtualM[i][j] = -1 ;
test = true ;
break;
}
else if(c=='c' && virtualM[j][i] == 0) {
images[j][i].setImageResource(R.drawable.red_0);
virtualM[j][i] = -1 ;
test = true ;
break;
}
}
else if(a==-1)
{
if(c=='d' && i==j && virtualM[i][j]==0) {
images[i][j].setImageResource(R.drawable.red_0);
virtualM[i][j] = -1;
test = true;
break;
}
else if(c=='w' && i+j==2 && virtualM[i][j]==0) {
images[i][j].setImageResource(R.drawable.red_0);
virtualM[i][j] = -1;
test = true ;
break;
}
}
}
if(test)
break;
}
}
@Override
public void onClick(View v)
{
setVirtualMatrix(v);
if(gameOver()==1)
Toast.makeText(this,"Player 1 Win",Toast.LENGTH_LONG).show();
else if(gameOver()==2)
Toast.makeText(this,"Player 2 Win",Toast.LENGTH_LONG).show();
else if(gameOver()==3)
Toast.makeText(this,"Draw",Toast.LENGTH_LONG).show();
if(gameOver() != 0)
{
for(int i=0;i<3;i++)
for(int j=0;j<3;j++)
{
images[i][j].setOnClickListener(null);
}
}
}
public void restart(View v)
{
for(int i=0;i<3;i++)
for(int j=0;j<3;j++)
{
images[i][j].setImageResource(R.drawable.shapes);
virtualM[i][j] = 0;
}
player = true ;
for(int i=0;i<3;i++)
for(int j=0;j<3;j++)
{
images[i][j].setOnClickListener(this);
}
game_mode = "multi/";
}
public void changeMode(View view) {
restart(view);
if(view.getId()==bsystem.getId())
game_mode = "vsSystem" ;
else if (view.getId()==bsystem1.getId()) {
game_mode = "vsSystem1/";
AlertDialog.Builder alertBuilder = new AlertDialog.Builder(MainActivity.this);
alertBuilder.setTitle("Level");
alertBuilder.setMessage("VS IA System Level");
alertBuilder.setPositiveButton("OK", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
}
});
alertBuilder.show();
}
}
}
At this stage our game it works as expected with three modes to play:
- In the normal mode when two-player play together the first one choose the X symbol. Then the second choice the 0 symbols.
- The Vs System mode when a player plays vs its computer, it’s a simple round when you want to play solo.
- The Vs artificial intelligence system this the most difficult mode, it’s an advanced mode to get grow in this game.
- This mode is dedicated to someone good at the Tic Tac Toe game, you can practice more with simple mode and then passe the AI model to improve yourself in this game.
Conclusion:
Thank you for reading this post, you can find more posts on elgarnaoui.com.
To support us share this post with your friends on social media and subscribe to our social media pages.
Feel free to comment if you have any issues or requests to us.